This was my first time to participated in Codeforces (http://www.codeforces.com/) , an online programming contest site.
This is a link explaining rules about the contest : http://codeforces.com/blog/entry/456
During the contest, I made a newbie mistake of resubmitting the same solution twice because I didn't understand what "judgement failed." meant. I thought it was something like "compilation error." Only after resubmitting my solution did I learn that "judgement failed" meant "there is something wrong with our server. Please wait a while we fix this issue." I lost about 100 points for that mistake.
But other than that, things went okay.
I solved three problems out of five - A , B, and C.
Here is the link to problem statement : http://codeforces.com/contest/149
And here is the link to my blog : : http://sk765.blogspot.com/
Problem A — A Business trip
Algorithm
Our main character, Petya, wants to water her plant as few time as possible while still achieving the goal of making her plant grow more than or equal to k centimeters.
We can use a greedy approach. Follow below steps
1. Sort month by how many centimeters they can make a flower grow in ascending order.
2. Pick the month with the largest growth value, and add it to variable named "sum".
3. Repeat procedure 2 until value of "sum" >= k
Source code
- #1 Each boy plays for exactly one team (x + y = n).
- #2 The sizes of teams differ in no more than one (|x - y| ≤ 1).
- #3 The total football playing skills for two teams differ in no more than by the value of skill the best player in the yard has. More formally:
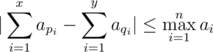
codeforces.com/blog/entry/3864
I searched for Editorial, and nothing showed up. I probably should've searched for Tutorial. And I didn't know only one person is allowed to post a review on a contest.
My solution with explanation for C
very bad kachestvo of razbor please redo it!