Sometimes you can meet interactive problems on programming contests (including Codeforces).
In problems of this type, the input data given to your program may be not predetermined but is built specifically for your solution. Jury writes a special program — interactor, such that its output is tranferred to the input of your solution, and the output of your program is sent to interactor’s input. In the other words, your solution and the interactor exchange the data and my decide what to print based on the "history of communication".
When you write the solution for the interactive problem it is important to keep in mind that if you output some data it is possible that this data is first placed to some internal buffer and may be not directly transferred to the interactor. In order to avoid such situation you have to use special flush
operation each time you output some data. There are these flush
operations in standard libraries of almost all languages. For example, in C++ you may use fflush(stdout)
or cout << flush
(it depends on what do you use for output data — scanf/printf
or cout
). In Java you can use method flush
for output stream, for example, System.out.flush()
. In Python you can use stdout.flush()
. In Pascal you can use flush(output)
.
There are some features for interactive problems:
- Input/output in interactive problems works much slower than in usual problems — try to use scanf/printf instead of cin/cout in С++, BufferedReader/PrintWriter in Java and etc.
- Usually, manual testing of the solutions for interactive problems much more difficult, because the participant needed to be in the role of interactor during testing.
- The "Custom invocation" tab does not know about the interactor for the problem, so you can't fully testing your solution.
- Sometimes on the Codeforces Rounds interactive problems will use. In this case the fromat of tests for hacks will described in the statements of the problems.
- Output endl in cout in C++ performs flush operation automatically.
Let's consider the following interactive problem. You can try to solve it here http://codeforces.com/gym/101021/problem/1
Problem
Guess the number
Statement
In this problem there is some hidden number and you have to interactively guess it. The hidden number is always an integer from 1 and to 1 000 000.
You can make queries to the testing system. Each query is one integer from 1 to 1 000 000. Flush output stream after printing each query. There are two different responses testing program can provide:
- string
<
(without quotes), if the hidden number is less than the integer in your query; - string
>=
(without quotes), if the hidden number is greater than or equal to the integer in your query.
When your program wants to guess the hidden number, print string ! x
, where x is the answer, and terminate your program immediately after flushing the output stream.
Your program is allowed to make no more than 25 queries (not including printing the answer) to the testing system.
Input
Use standard input to read the responses to the queries.
The input will contain responses to your queries — strings <
and >=
. The i-th string is a response to the i-th your query. When your program will guess the number print ! x
, where x is the answer and terminate your program.
The testing system will allow you to read the response on the query only after your program print the query for the system and perform flush
operation.
Output
To make the queries your program must use standard output.
Your program must print the queries — integer numbers xi (1 ≤ xi ≤ 106), one query per line. After printing each line your program must perform operation flush
.
Each of the values xi mean the query to the testing system. The response to the query will be given in the input file after you flush output. In case your program guessed the number x, print string ! x
, where x — is the answer, and terminate your program.
Solution
Of course, this problem can be solved using binary search. Here is an example of the C++ solution:
#include <cstdio>
#include <cstring>
using namespace std;
int main() {
int l = 1, r = 1000000;
while (l != r) {
int mid = (l + r + 1) / 2;
printf("%d\n", mid);
fflush(stdout);
char response[3];
scanf("%s", response);
if (strcmp(response, "<") == 0)
r = mid - 1;
else
l = mid;
}
printf("! %d\n", l);
fflush(stdout);
}
We wish you accepted solutions. Once again, you can solve simple interactive problem here http://codeforces.com/gym/101021/problem/1
can i use "ios::sync_with_stdio(false)" with cin/cout instead of scanf/printf in c++ or it's Better to Use scanf/printf ?
You can use cin/cout but still you should flush the output.
Okay , Thank You Errichto
It is said that it's slow, may it get TLE??
Sometimes it can. Even with using of "ios::sync_with_stdio(false)" cin/cout is slower than scanf/printf.
"endl" flushes automatically for you. cout<<endl is more or less the same as cout<<"\n", fflush(stdout) (already written by Mike, but I think that needs emphasizing).
I suspect that if you don't write
cin.tie(0)
,cin >> x
also flushes automatically.Do we need to flush cin?
No, we need to flush cout only because the output is buffered and not actually printed sometimes.
So, you do NOT need to flush cin
I just got an AC!(Comment link)
Was trying to find the error. "/n vs endl should be emphasized.
how would you test these kinds of problems on your local computer?
You can take a solution from the blog above and run it on your computer. The program will ask you queries and you should print responses
<
and>=
.Here is another interactive problem to try from (2012-2013 ACM-ICPC, NEERC, Moscow Subregional Contest):
J. Just Another Disney Problem
You don't need "return 0;" for interactive problems?
In C++
main
can be left without a return value at which point it defaults to returning 0.In hacks, how would the "output" of model/participant solution be shown?
For some problem like this or this it seems we can see the whole process (look at some submissions), but the example problem from this post just shows the hidden number and the number of queries.
In the problem above (from this blog) the input for hacking would be the hidden number. In the round today, the information about hacking will be in the statement.
It is very funny :D using binary search (the exact code of blog) with
L = 1, R = 1e6+6
got wrong answer on test 6, butL = 1, R = 1e6
got Accepted!L = 1, R = 1e6+6
: submissionL = 1, R = 1e6
: submissionYour program must print the queries — integer numbers xi (1 ≤ xi ≤ 10**6), one query per line.
Range of the input numbers is 1 to 1000000. That's why.
Why I always can't see other's people submission by links like this http://codeforces.com/gym/101021/submission/18299998?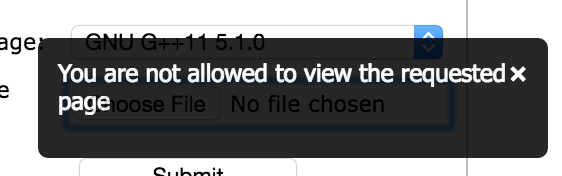
Here's another interactive problem. https://www.codechef.com/MAY16/problems/CHBLLS Anyone interested may take a look. My first interactive problem. I enjoyed the solution but didn't like that flushing part somehow :v Even if someone understands the logic, he has the chance to get a wrong verdict for the syntax.
My solution gets
Idleness Limit Exceeded
, even though I flush the buffer. Where do you think the problem is?18300219
UPD: I've found the error! I should've printed one query per line.
hello,
any one could tell me why this code gets TLE on test 2 whilst the same code in the blog gets AC which just differs in reckoning of
mid
, while I make r equals to 1e6+1 to evade that .any help would be highly appreciated
if
l+1==r
then your code runs forever.If response were <= and > then mid=(l+r)/2 would work, right?
It doesnt work beacuse of overflow. For instance if l=9 and r=10, (l+r)/2 will be 9 So mid doesnt change and l<r is true forever.
This is why the above code does (l+r+1)/2
mid = (l+r+1)>>1
why in this round ? you could use it in the educational first
Getting Idleness limit exceeded on test 1 in the first given problem. Here is my code. How can I fix it?
Read the task.
Read the example code by Mike.
ohh... I missed the example code. I am not used to this system. why this???
Because it is very interesting and new for CF community
please clear me 2 things.
the given output is my input
I need to use flash after every output.
right? anything else?
I have not idea about it. so please clear me.
Your program must send requests(input) to interactor,and interactor answers for request(output). If you test your code local, you should answer for request by using input.
You should use flush for correct interactive work.
Should I flush the buffer after each and every test case or once after the entire program is over?
An Educational Round on this type of Problems would be better to understand the Submit / Hacking System .
Mike don't get me wrong it sounds like this contest is gonna be brilliant but couldn't you post this earlier like a day or two earlier.
or you could have tried it with educational rounds first like Deathly_Hallows said.
Nevertheless I wish happy coding for everyone and high ratings good luck.
:)
hi.. actually users facing interacting problems for the first time may experience significant drop in ratings.. so please make the announcements a bit before or we can have a testing round or educational for such purposes.. its nice that codeforces comes up with newer challenges.. thanks.. :)
what will be the verdict for making more than 25 queries???!!
should be WA but you can check it.
Wrong Answer
The example section looks a bit misleading: why ">=" / "<" are output instead of input? And I think it'd be better to arrange them chronologically, like this.
TL;DR — you are right, but it isn't important, right?
You are right that it's misleading and should be other way around. It's my mistake. It will be displayed correctly in the round (with ">=" as input in this case).
This is not TL;DR, it's FL;DU (Foreign language; didn't understand) :D
Actually I don't get your comment ;p
Did I write something not clear? If yes then I will rewrite it.
The link which cgy4ever provided is FL;DU not TL;DR :D
So, what do you think about the format we used? There were tables in the Notes section. Any better solution?
It looks perfect. (The only issue may be that we can't copy-paste since it is a picture, but for that specific problem it is ok.)
Huh. I've just realized that it's impossible to copy-paste. Strange — because it's not a picture, it's an HTML table.
Why's that
"r = mid - 1"
and"l = mid"
gets AC and"r = mid - 1"
and"l = mid + 1"
gets WA? Can someone explain it?for mid if response is < then hidden number is less than mid so r = mid - 1 if response is >= then hidden number is mid or greater than mid so l = mid
Got it, thank you
You can do
l = mid + 1
andans = mid
. And print ans in the end.I recommend not to penalize errors on 1 test for the interactive problem as many people might get confused initially.
Errors on 1st test are not penalized even for other problems
Nice to know, thanks.
I guess Input and Output were mutually misplaced?
I need to Input '<=' and '>' and let computer to guess and Output the number?
Input and Output are displayed swapped in this problem, sorry. It will be displayed correctly during the round.
All the problems will be "Interactive"? All 5 from today's contest?
No.
thanks
only 1 out of 5 !
thanks
I don't think so because it's not quite easy to solve an interactive problem especially with the difficulty of A and B div 2.
Would it be possible to hack an interactive problem?
http://codeforces.com/blog/entry/45307?locale=ru#comment-298510
Sometimes on the Codeforces Rounds interactive problems will use. In this case the fromat of tests for hacks will described in the statements of the problems.
All the problems will be interactive?
http://codeforces.com/blog/entry/45307?#comment-298587
If you have a question
{
}
Doesn’t
std::endl
flush the output buffer in C++ and doesn’tstd::cin
flush it as well? I’m pretty much surprised that 18314320 fails while 18315037 gets accepted.You have different loop condition, lol
Oops, sorry :D
That’s what happens when one doesn’t write contests for a long time!
Thanks, but I made mistake in the understanding of the task.
I should to go on a new line after flush.
I mean:
cout<<flush<<Something<<endl;
Actually,
flush
beforeSomething
just flushes an empty buffer, so it can be omitted.It's very important to specify whether the system actually "hides" a fixed integer before interaction starts or the interactor can change the number (or any other information, in general) during testing in a way consistent with previous answers. In the former case, some probabilistic solutions to some problems can pass. In the latter, they won't.
As far as I can see, the only place in the example problem which mentions it is "it's fine to guess — if you guess correctly".
The second sample clearly shows it. But yes, I agree that it should also be written in the statement. We adjusted this problem in hurry and it isn't perfect (we decided to show the guide in the day of the contest).
Also, in some problems the system may be smarter/malicious and answer to more likely fail a participant. But we didn't want it in "prime 100" problem.
Maybe there should be another status for protocol failure (e.g. query limit exceeded)? Even Runtime Error would be much better than WA.
I don't see a reason. In standard problems there shouldn't be "WA because your answer is too small" or "WA because an edge you printed doesn't exist". So, why should we get extra info here?
But there is in fact presentation error if you violate output protocol (e.g. give less integers than needed)
I'd really like to see query limit as PE than as WA, because you're actually violating protocol by printing more queries than it was possible.
Are you sure about PE? I thought that there is no PE in CF rounds — source.
And I don't think it's possible to create some exact rules what is PE and what is WA. For example, a participant should print the number of vertices satisfying something — then what if he/she prints - 1 or n + 1? Should it be WA or PE? It's only an example.
Please Help Me Out ..
I am trying to solve the Interactive Problem given in the above Link as there may be an Interactive Problem in today's Round . I have submitted a Code for practice several times. But Its showing WA at Test 1 . Don't know why .
Problem: http://codeforces.com/gym/101021/problem/A
Here is My code: http://pastebin.ubuntu.com/23172422/
It'd be very helpful if you please point me out the erroneous point of my Code .
Thanks in Advance .
Is writing
fflush(stdout);
at the end of main() necessary or redundant?You need to use 'flush' operation only after every print.
I meant, what about the last print. eg: in the code given in the blog,
printf("! %d\n", l); fflush(stdout);
is fflush(stdout) needed here?
Yes, you need.
Actually not. At the shutdown of the program stdout is automatically flushed. Though it is a good practise in an interactive problem to flush after any print because it doesn't make anything worse but prevents you from forgetting about some important flush.
More details.
In Python 3, print() is a function and it has an optional parameter "flush" with a default value false. So you can just set it to true when needed to flush the output stream. print("blah blah", flush = True)
40769854
40769788
In real test, the print works even with "flush = False",
I don't know why..
Any idea what this error means
wrong output format Unexpected end of file - token expected
submission
You are outputting nothing.
Yeah, I just realized my code skips all ifs. I am new to this type of problem.
Even this works
cout.flush();
Can some one explain why this
It isn't equivalent. One rounds up, the other rounds down. Consider what happens when l + 1 = r.
In the just concluded contest, I couldn't accept the input in python 2.
Problem: https://codeforces.com/contest/1011/problem/D
My simple solution:
The verdict: Idleness limit exceeded.
Any help is appreciated.
have you tried reading the post you comment (or problem description)? You need to flush each time you print something. 40814436
Yes sir, I've got my answer. Thanks a lot.
Why is this solution wrong?
/* _______ _______ _______ _______ _______ _______ ( ____ ( ____ ( )( ____ )( ____ ( ____ ) | ( \/| ( \/| () () || ( )|| ( \/| ( )| | (_____ | (__ | || || || (____)|| (__ | (____)| (_____ )| ) | |(_)| || _____)| ) | __) ) || ( | | | || ( | ( | (\ (
/_) || (__/| ) ( || ) | (____/| ) \ __ _______)(_______/|/ ||/ (_______/|/ __/
_______ _________ ______ _______ _ _________ _______ ( ____ __ /( __ \ ( ____ ( \ __ /( ____ \ | ( \/ ) ( | ( \ )| ( \/| ( ) ( | ( \/ | ( | | | | ) || ( | | | | | (_____ | ) | | | | | || ) | | | | (_ ) | ( | | | | ) || ( | | | | ) | | ) ) (| (__/ )| (____/| (____/___) (___/____) | |/ _______/(______/ (_______/(_______/_______/_______)
*/
include <bits/stdc++.h>
using namespace std;
define ll long long
define N 100005
define ld long double
define M 1000000007
define dd double
define rep(i,a,n) for(int i = a; i < n; i++)
define sep(i,n,b) for(int i=n-1;i>=b;i--)
define pb push_back
define mp make_pair
define Mll map<ll,ll>
define clr(x) x.clear()
define sz(x) ((int)(x).size())
define F first
define S second
int main() { int repeat=25; string tans; int start=0; int end=1000000; int mid=(start+end)/2; cout << mid << "\n"; cin >> tans; map<int,int> v; while(repeat--){ if(tans=="<"){ v[mid]=10; if(v[mid]==10 && v[mid+1]==11){ cout << "! " << mid+1 << endl; fflush(stdout); return 0; } start=mid; mid=(start+end)/2; cout << mid << "\n"; fflush(stdout); cin >> tans; } else if(tans==">="){ v[mid]=11; if(v[mid]==11 && v[mid-1]==10){ cout << "! " << mid << endl; fflush(stdout); return 0; } end=mid; mid=(start+end)/2; cout << mid << "\n"; fflush(stdout); cin >> tans; } } return 0; }
// >=
Why is this solution wrong?
http://codeforces.com/gym/101021/submission/47026197
you messed up with "<" and ">="
Yes. I realized. Thanks. But this still won't work due to some other reason I don't understand.
Can someone explain why "L" is printed and not MID? And also when to print L , MID and R in binary search problems?
Errichto can you please explain this?
They are all equal at the end.
Are mid and l equal in all cases in this problem?
They are.
Ok I understood , thank you so much.
Who wants to add new Interactive problen tag?
here latest one
This one from July Long 2019:- https://www.codechef.com/JULY19B/problems/GUESSPRM
Can I use "system("cls");" instead of "fflush(stdout);" in C++?
80301018 I am getting WA on test 6. I don't know why. can anyone help?
If i solved one of them would it be worth for rating?
Why do I still get the Idleness error despite of using flush after output (I have tried both flush after each output and just after the last output).
Can I use endl to achieve the effect of fflush(stdout)?
Why this code is falling on test case 1
You forgot space after !
cout << "! " << ans <<endl;
It should be format, not fromat, thought this isn't a big problem lol.